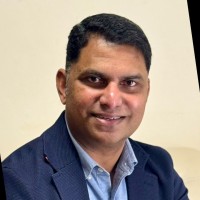
An Azure Function is a serverless computing service provided by Microsoft Azure that allows you to run code in response to events without having to manage the infrastructure
It’s designed to simplify and speed up the development of event-driven applications by automatically handling the underlying resources, scaling, and management.
Azure Functions can be triggered by a variety of events, such as HTTP requests, messages from Azure Service Bus, changes in Azure Cosmos DB, blob storage updates, timers, and more.
Use Cases
- Data Processing: Process data in real-time when new data is added to a storage account, database, or IoT hub.
- Event-driven Applications: Trigger functions when specific events occur, such as user registration or order completion.
- Microservices: Build small, focused pieces of functionality that interact with each other as part of a larger application.
- Scheduled Tasks: Execute code at specified intervals, similar to a CRON job.
- API and Webhooks: Use Azure Functions to build lightweight APIs or handle webhook calls
Implementation Example - Step By Step
Story : Implement a time trigger function to remove unsubmitted timesheet for a given number of days
Step 1:
Create a new Azure Function Project from Visual Studio 2022 (Data.Function.csproj)
Step 2:
Add the following sample code (RemoveUnsubmittedTimesheets.cs)
using System.Threading.Tasks;
using Data.Function.Services.Interfaces;
using Microsoft.Azure.Functions.Worker;
namespace Data.Function
{
public class RemoveTimesheets
{
private readonly IRemoveUnsubmittedTimesheetsService _removeUnsubmittedTimesheetsService;
public RemoveUnsubmittedTimesheets(IRemoveUnsubmittedTimesheetsService removeUnsubmittedTimesheetsService)
{
_removeUnsubmittedTimesheetsService = removeUnsubmittedTimesheetsService;
}
[Function(nameof(RemoveUnsubmittedTimesheets))]
public async Task Run([TimerTrigger($"%{nameof(RemoveUnsubmittedTimesheets)}TriggerTimer%")] TimerInfo myTimer)
{
await _removeUnsubmittedTimesheetsService.RemoveTimeSheet();
}
}
}
Step3:
Configure the function to run (local.settings.json)
{....
"Values": {
"AzureWebJobsStorage": "UseDevelopmentStorage=true",
"FUNCTIONS_WORKER_RUNTIME": "dotnet-isolated",
"RemoveUnsubmittedTimesheetsTriggerTimer": "0 */3 * * * *",
// NOTE: Disabling functions disables the triggers, but still allows them to be invoked manually
// Use the .http file in the project to trigger functions.
// To invoke functions manually, run:
// Invoke-RestMethod -Method Post -ContentType "application/json" -Body "{}" -Uri http://localhost:7071/functions/<function-name>
// OR
// curl.exe --json "{}" http://localhost:7071/functions/<function-name>
"AzureWebJobs.RemoveUnsubmittedTimesheets.Disabled": true,
// To alter local logging level, this Function log level must match or be lower than the lowest level defined in Logging:LogLevel in this settings.json/appconfig
"AzureFunctionsJobHost:logging:logLevel:Function": "Trace"
},
}
Step 4:
Implement the 'RemoveTimeSheet' service method in your choice to remove data with logging, auditing etc..
Step 4:
Run function using set the project as start-up and send a http request. Add the file (Data.Function.http) to the project.
@Endpoint=http://localhost:7071/functions
### Invoke function to remove unsubmitted timesheets
POST {{Endpoint}}/RemoveUnsubmittedTimesheets
Content-Type: application/json
{}